Selenium Automation Testing
Selenium was originally developed by Jason Huggins in 2004 as an internal tool at ThoughtWorks.
Selenium is an open source. Originally It provides a recording and playback for functional tests. It consists of Selenium IDE, Selenium Grid, Selenium RC and Selenium Web Driver. Selenium has web browser compatibility, that is it can work on Chrome, Firefox, Edge, Opera, htmlunit, etc. Selenium also has multi system compatibility, it can work in Windows, Mac, and Linux. Selenium works well with multiple programming languages, such as Java, C#, Python, Ruby, and PHP. Selenium is mainly used to test web browsers for use interface functional tests. Selenium has its own methods and commands to operate on web elements for implementation of web browser testing. We will discuss selenium automation testing as following aspects: basic of Selenium and set up Selenium-Java-Maven Test Projects.
I. Selenium WebDrivers
Selenium WebDriver is a programming Interface with which selenium library interacts and then WebDriver performs actions on web elements. Interacting with WebDriver we can create test cases and run test cases. WebDriver supports various programming languages such as Java ,C#, Python, etc.
As above picture, we can code a program using Selenium Library and run the program which call Selenium WebDriver and then WebDriver interacts with web browsers and WebDriver performs actions on web elements of a web site. Also, from the picture we know if we want to set up selenium automation testing first we may do as following:
Select Selenium Library, take Java-maven for example, add the following items in POM File:
<dependency> <groupId>org.seleniumhq.selenium<groupId> <artifactId>selenium-java<artifactId> <version>4.8.1</version> <dependency>
Set up driver instance if we use WebDriver for Chrome:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.webdriver.chrome.option;
System.setProperty("webdriver.chrome.driver","/path/to/chromedriver"); //indicate what is driver and where is driver.
ChromeDriver driver = new ChromeDriver(); // Create a new driver instance
Or we can use,
ChromeOption option = new ChromeOption();
ChromeDriver driver = new ChromeDriver(option); // Create a new driver instance with option
II. Selenium Locators
What are locators? If you want to find a person, you may use user address, phone number, email address, selenium how to find web elements, it uses locators. There are useful locators in Selenium:
1. Using Id locates web element which it is matched with search value, for example driver.findElement(By.id(“webElement”))
2. Using class locates web element which class is matched with search value, for example driver.findElement(By.class(“webElement”))
3. Using name locates web element which name is matched with search value, for example driver.findElement(By.name(“webElement”))
4. Using xpath locates web element which xpath is matched with search value, for example driver.findElement(By.xpath(“webElement”))
5. Using link text locates web element which link text is matched with search value, for example driver. findElement(By.linkText(“webElement”))
6. Using link text locates web element which partial link text is matched with search value, for example driver. findElement(By.linkText(“webElement”))
How to get a locator of web element? I use Chrome for example, open web site in Chrome(https://www.admlucid.com/WebElement), right-click on a web element -> Inspect -> right-click -> Copy(Copy Element, Copy Selector, Copy Xpath)
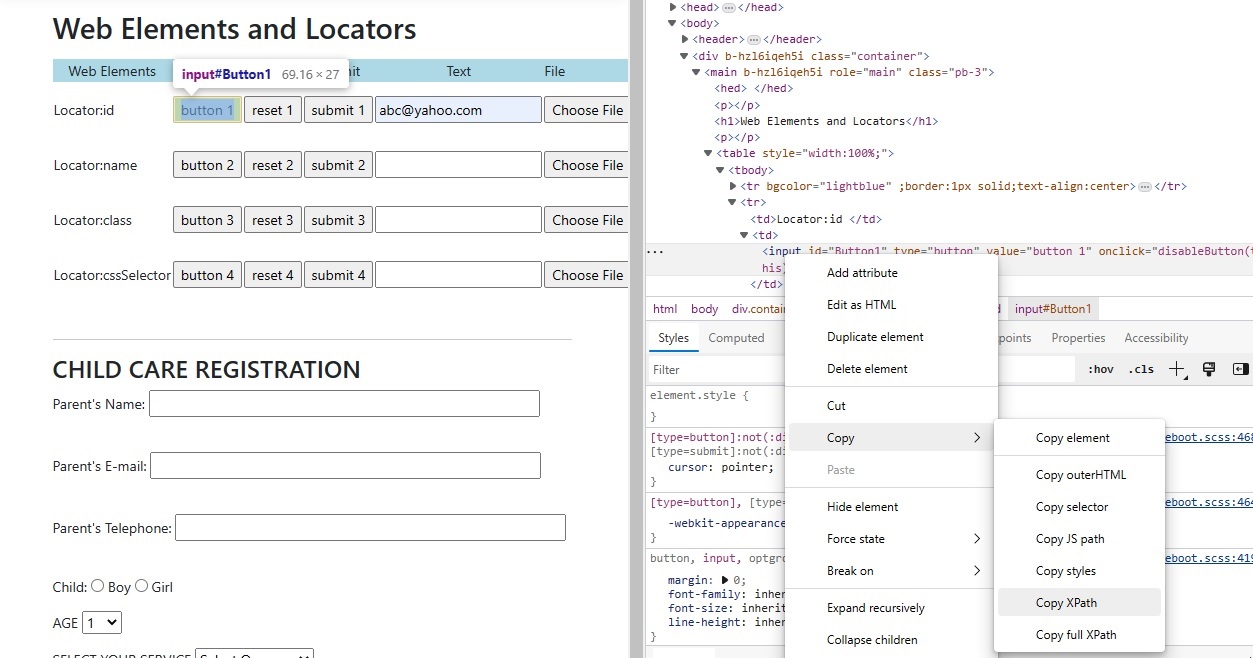
III. Selenium Commands
Selenium has commands to operate web elements:
driver.findElement(By.id(“webElement”)): selenium first locates a web element using this command.
click(): selenium finds button or text link and click on it.
getText(): selenium finds a web element and gets text from it.
clear(): selenium finds text box or text area and clears its containing text.
sendKey(): selenium finds text box or text area and types text in it.
isExisted() or isPresented(): selenium assures if a web element exists.
isEnabled(): selenium assures if a button is enabled.
isSelected(): selenium check if radio is selected.
isChecked(): selenium check if checkbox is checked.
getCurrentUrl(): selenium gets driver URL.
getTitle(): selenium gets web page title.
Select: selenium select item from a dropdown list
switchTo(): in selenium testing sometime there is more than one window to open(two windows), switchTo() can switch among the windows.
navigate(): driver navigates to a web site.
getWindowHandle() or getWindowHandles(): Selenium WebDriver can handle multiple windows. If the window name is not known, we can use it to obtain a list of known windows.
Waits: Sometime web elements of page are not loaded, selenium testing needs to wait for web elements’ coming up and do operations on them, in the case we need to use Wait. There are three kinds of Wait in Selenium, Explicit wait, Implicit wait and FluentWait.
Close(): Close tab/window
Quit(): Quit driver.
IV. Use Page Object Model to design Test Application of Selenium-Java
Is a design pattern, in automation testing we can create Object Repository for web elements. Our test contains Login, Golf Course and Book; we design Objects as three page-classes of Login, Golf Course and Book to store their web elements. The model can reduce code duplication and improve test maintenance. The page-classes will handle web elements and methods.
V. Test Framework of Selenium-Java-Maven
When you design Test Framework, you may think about code reuse, application reboots, easily integrating features to be tested, configuration to handle environment changes and nice reports. This is an example of our test framework:
1. Web elements.
2. Manage web elements.
3. Mange testing browsers.
4. Re Using functions or methods.
5. Configuration: URL, user information, web driver’s location, exporting reports
6. Nice reporting format with screenshots, user can easily understand every step of running test cases.